Top Front-End Development Resources
I've combed through my browser history to pinpoint the resources that I turn to daily. I've also included a selection of the most interesting ones I've stumbled upon, which you can integrate into your side projects to speed up development.
Enjoy! 🚀
UI Components/Styling 🎨
- Tailwind Variants: a notch above
class-variance-authority
for me because it supports multipart components and responsive variants.
import { tv } from "tailwind-variants";
const button = tv({
base: "font-medium bg-blue-500 text-white rounded-full active:opacity-80",
variants: {
color: {
primary: "bg-blue-500 text-white",
secondary: "bg-purple-500 text-white",
},
size: {
sm: "text-sm",
md: "text-base",
lg: "px-4 py-3 text-lg",
},
},
compoundVariants: [
{
size: ["sm", "md"],
class: "px-3 py-1",
},
],
defaultVariants: {
size: "md",
color: "primary",
},
});
return <button className={button({ size: "sm", color: "secondary" })}>Click me</button>;
It's a real timesaver for devs looking to bypass the design hustle.
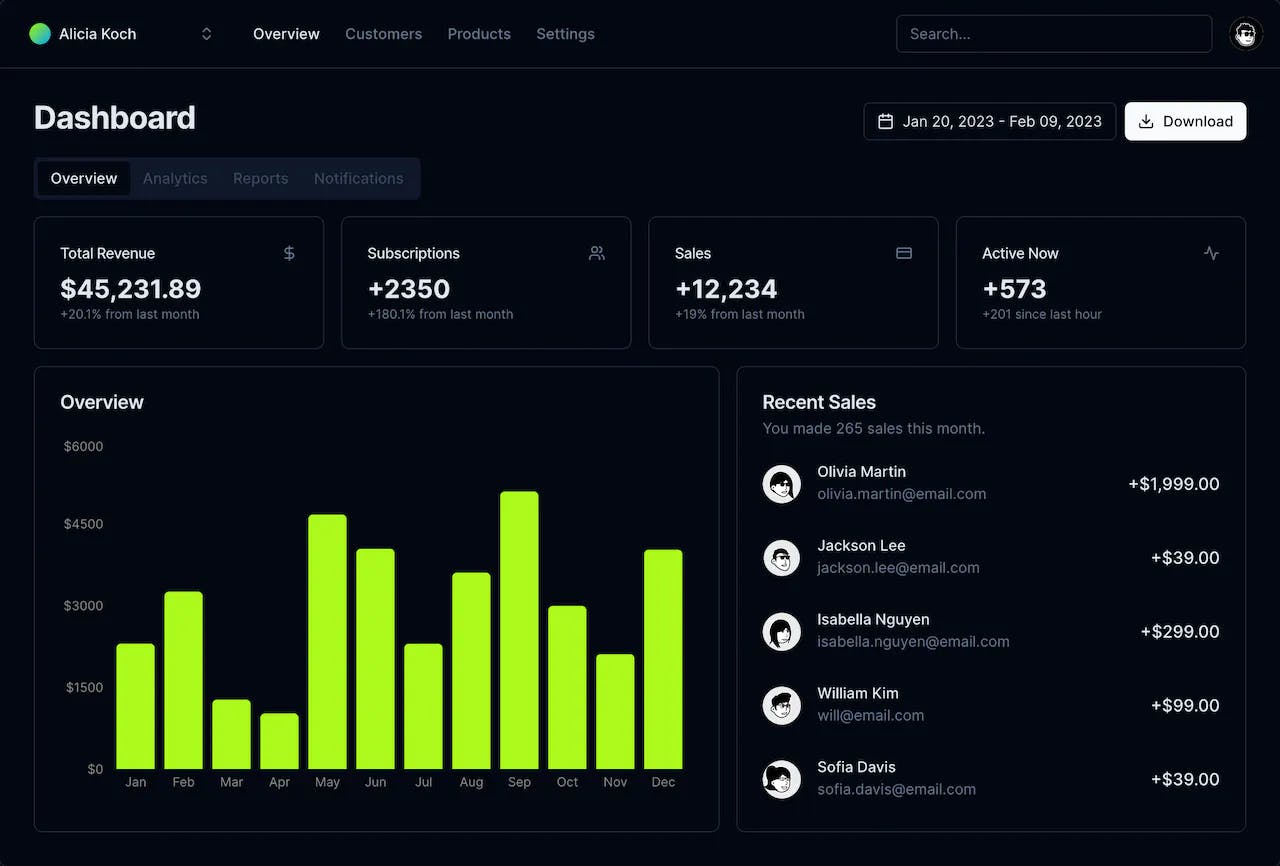
-
React Wrap Balancer: a Vercel package that makes titles more readable.
-
Chakra-UI: Chakra holds a special place in my heart ❤️. Its only hiccup is client-side component reliance. Some packages made by the same team:
Read more about their future: Chakra, Panda and Ark - What's the plan?
- React email: ui components for email.
Supports Tailwind. Tested on popular clients such as Gmail, Apple Mail, Outlook, etc.
- whatamesh: Stripe-inspired gradient animation. Incorporate this into your next side project!
- cmdk: unstyled command menu for React.
Animations 🔥
- Framer Motion
- AutoAnimate: add animations with a single line of code.
import { useAutoAnimate } from "@formkit/auto-animate/react";
function MyList() {
const [animationParent] = useAutoAnimate();
return <ul ref={animationParent}>{/* 🪄 Magic animations for your list */}</ul>;
}
-
React Toggle Dark Mode: a cool theme switcher. I'm using it on this blog! ☝️
Forms 🔠
- React Hook Form
- Zod: TypeScript-first schema validation with static type inference.
Use these two libraries together to create type-safe forms.
import { useForm } from "react-hook-form";
import { zodResolver } from "@hookform/resolvers/zod";
import * as z from "zod";
const schema = z.object({
name: z.string().min(1, { message: "Required" }),
age: z.number().min(10),
});
const App = () => {
const { register, handleSubmit } = useForm({
resolver: zodResolver(schema),
});
return (
<form onSubmit={handleSubmit(d => console.log(d))}>
<input {...register("name")} />
<input type="number" {...register("age", { valueAsNumber: true })} />
<input type="submit" />
</form>
);
};
Authentication 🧑
- Clerk: easy to set up authentication and user management. Comes with pre-styled components like
<SignInButton />
and<OrganizationSwitcher />
Web3 💎
Tools 🛠️
-
GitHub Copilot: coding companion for auto-completions and more.
-
Measuremate (chrome extension): measure distances between elements on webpages.
-
Gyazo: record new videos and screenshots instantly.
Other
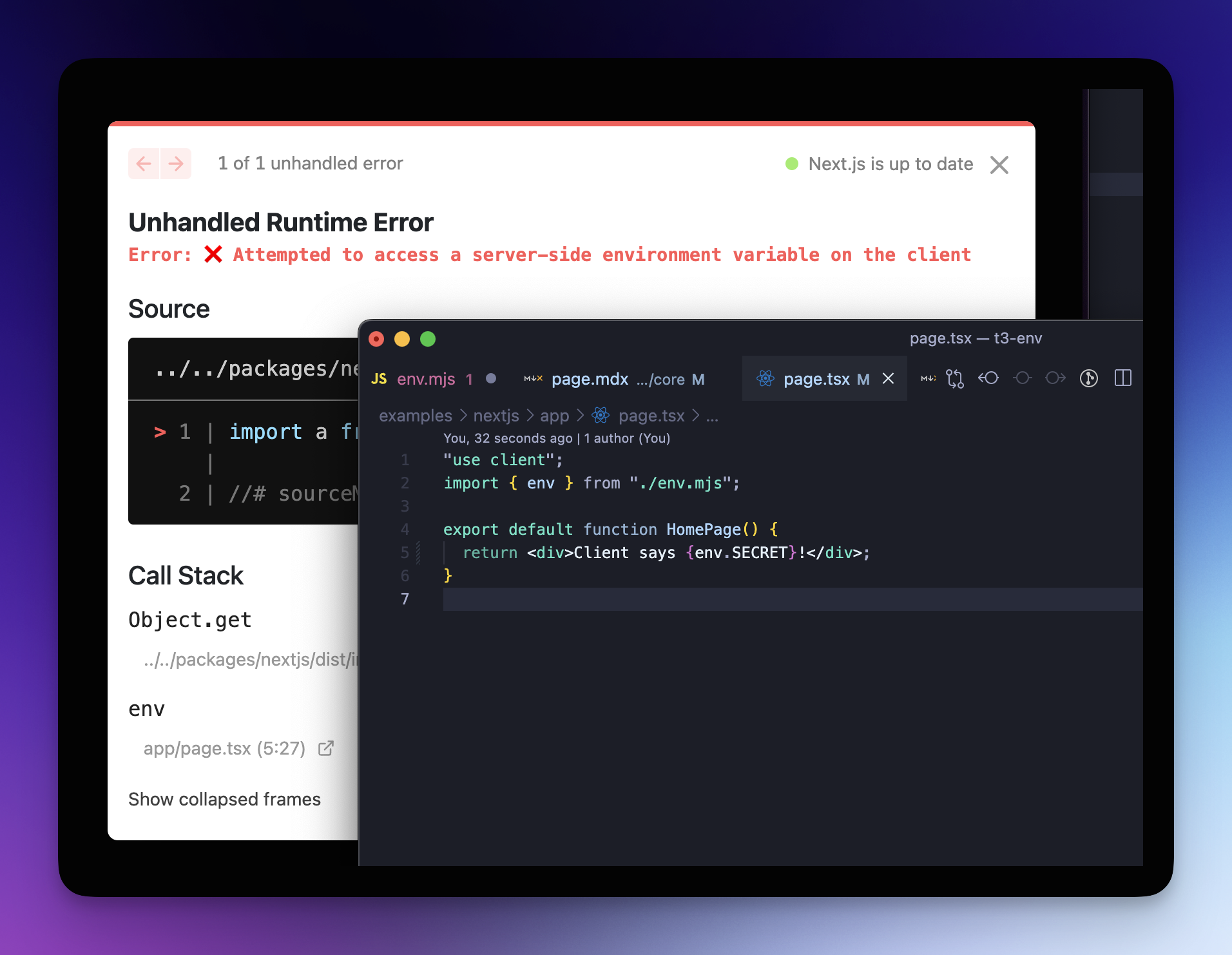
- Vercel's
ai
npm package: a library for building AI-powered streaming text and chat UIs.
const prompt = "Count to 10";
const response = await openai.chat.completions.create({
model: "gpt-3.5-turbo",
messages: [{ role: "user", content: prompt }],
});
- Drizzle: headless TypeScript ORM with a head 🐲.
import * as schema from "./schema";
import { drizzle } from "drizzle-orm/...";
const db = drizzle(client, { schema });
const result = await db.query.users.findMany({
with: {
posts: true,
},
});
- Dribbble - design inspiration.
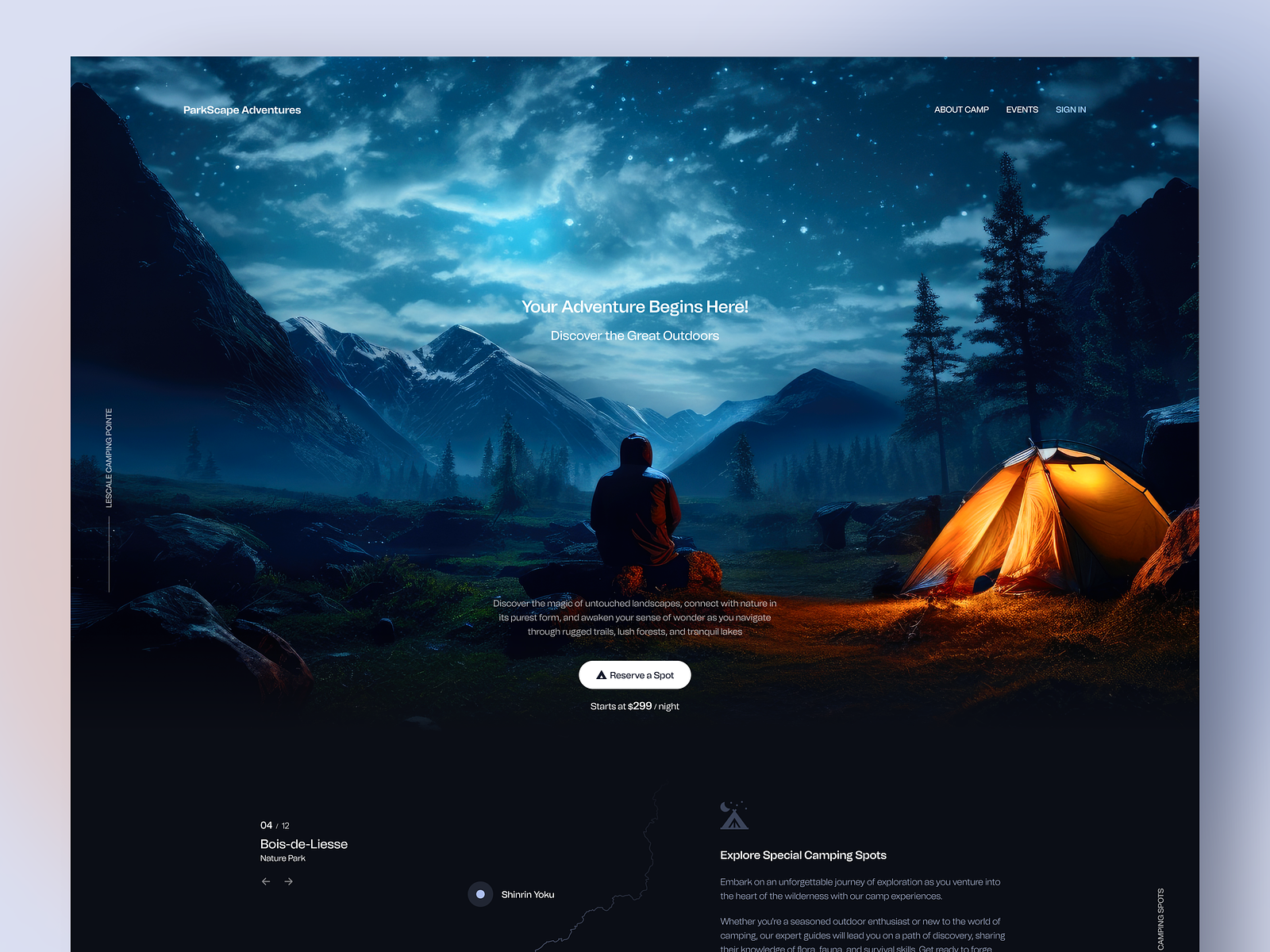
-
Next.js templates: templates that will speed up your initial setup. Btw, this blog was initialized from Next.js Contentlayer Blog Starter.
-
Bundlephobia: find the cost of adding a npm package to your bundle.
-
Mockaroo: generate up to 1,000 rows of realistic test data in CSV, JSON, SQL, and Excel formats.
Learning/reading 📖
-
Stack Overflow Survey 2023: the biggest engineering survey with over 90,000 respondents 🎉. If you're ever in doubt about what to learn next, this is the place to go. Other surveys:
- State of JavaScript
- State of CSS
- State of React: open at the time of writing.
-
React TypeScript Cheatsheet: useful typescript patterns.
-
Theo - t3.gg: a YouTube channel for everything engineering-related with a focus on the Next.js ecosystem.
-
Web Dev Simplified: a YouTube channel with a focus on frontend development.
-
The Framer Book: well documented
framer-motion
examples.
If you found this list useful, don't forget to 🔖 bookmark this page for future reference.
Happy coding! 🫶